iFrame & Web integration
This page will guide you through the implementation of BitLabs within an iFrame or web link.
iFrame integration
To provide the best experience for your users on your platform, we advise integrating BitLabs as an iFrame. BitLabs offers a lot of customization, which will allow you to configure it in a way, where it would blend into your style guide and website branding.
How to integrate via iFrame
Use the following code snipped to implement the BitLabs offer wall into your iFrame:
<iframe src="https://web.bitlabs.ai/?uid={USERID}&token={TOKEN}"></iframe>
In the above example, you need to replace the values of the following query parameters:
uid
: Replace the placeholder with the user ID you want to receive back from our callbacks in the [%UID%] parameter.token
: To identify the offer wall, you need to replace the placeholder with your offer wall token. You can find the token on the BitLabs Dashboard -> Apps -> Your app placement -> Integration -> App/API Token.
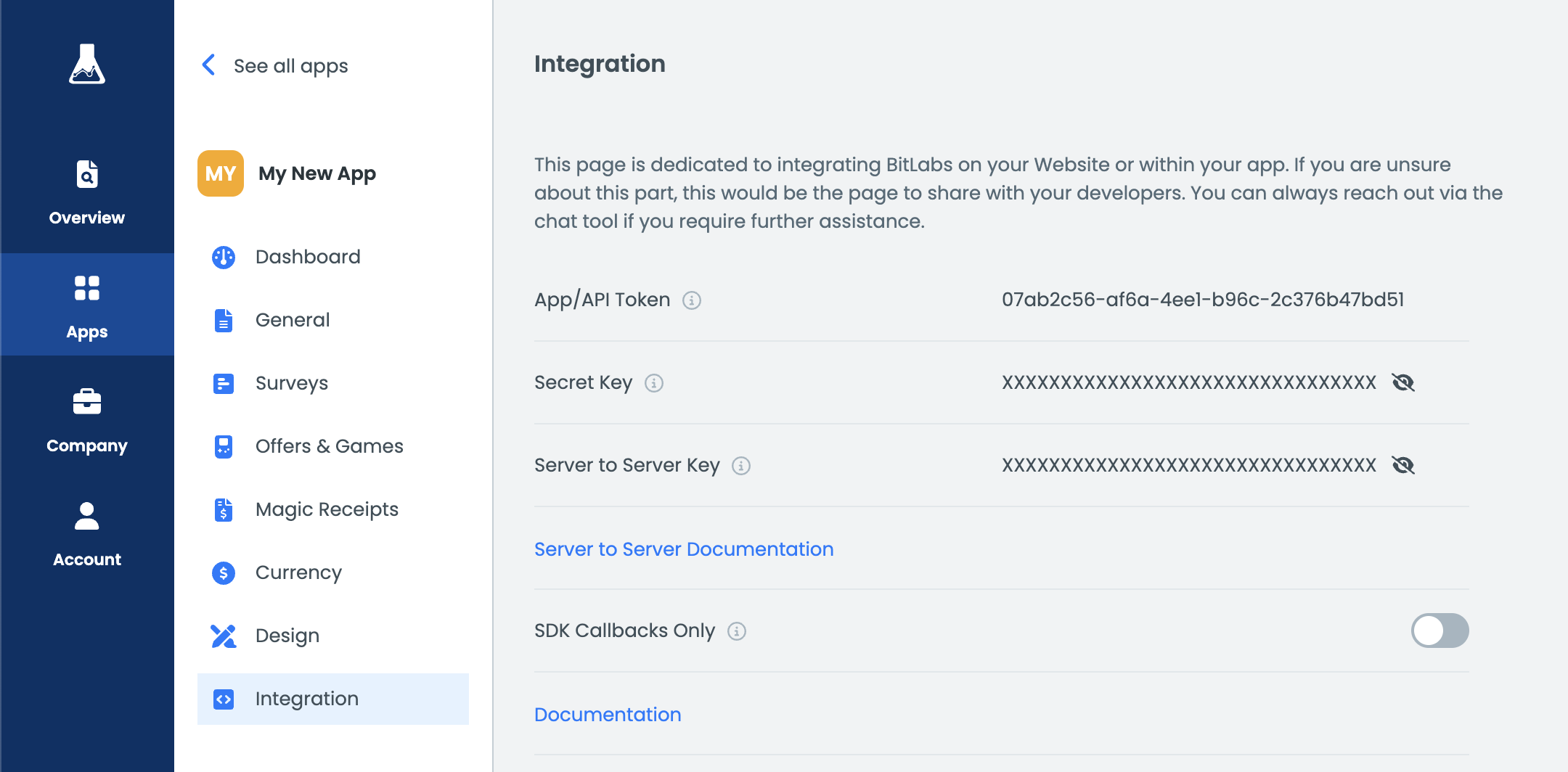
Link integration
Besides integrating via iFrame, you can also let users open the link to the offer wall directly. However, this is not recommended, since it will have downsides compared to the iFrame integration:
- Some opening query parameters do not get stored in locally. This means upon refreshing the offer wall page, some query parameters will get lost.
- In case the user clears their cache, the user id and token, which got stored locally, will be removed and the user will no longer be able to visit the offer wall unless they click on the opening URL with all the query parameters attached.
In general, we advice you to always integrate via an iFrame on Web.
How to integrate via a link
Similar to the iFrame integration, the offer wall will be opened via the web.bitlabs.ai URL. To open the offer wall in a new tab, you can use this JavaScript example snippet:
// This will open the offerwall in a new tab
window.open('https://web.bitlabs.ai/?uid={UID}&token={TOKEN}', '_blank')
Replace the placeholders according to what is explained in the iFrame integration guide.
Multiple offer wall placements
You can have multiple placements of the BitLabs offer wall on your website or app. It doesn't matter if you are using both mobile and web, the same app token can be used for all types of integrations, even if you want to use multiple different types of integrations at the same time.
In case you want to use one app token, but have placements for each demand type separately, that is also possible by using the display_mode
opening parameter. Learn more about opening parameters in the following section.
Opening parameters
To add more configuration options or information to your offer wall, we provide a variety of opening query parameters.
Mandatory parameters
These parameters are not optional and always have to be added to an opening link, otherwise the offer wall does not work.
Parameter | Possible values | Description |
---|---|---|
uid | String | Any string with a maximum length of 255 characters. Please do not use any of the following special characters: %, $, *, &, # |
token | App/API Token | The App/API Token you can find on the BitLabs Dashboard -> Apps -> Your app placement -> Integration -> App/API Token. |
Cosmetic parameters
These parameters change the appearance of the offer wall.
Parameter | Possible values | Description |
---|---|---|
currency | String or image URL | Overrides the currency string or icon |
custom_logo_url | image URL | Overrides the custom logo |
navigation_color | colors | Overrides the navigation color |
background_color | colors | Overrides the background color |
interaction_color | colors | Overrides the interaction color |
survey_icon_color | colors | Overrides the survey icon color |
theme | theme | Sets the color scheme the Offerwall should use |
Colors
The color parameters accept a hex color code like #ff00ff
or a linear-gradient.
The linear gradient needs to be in this format: linear-gradient(45deg, #ff0000 0%, #00ff00 100%)
. (the numbers can be changed).
Theme
The Offerwall can operate in two themes: Light and Dark. The theme is automatically set on startup based on the user's browser settings. However, it is possible to override the theme by using the theme
query parameter. Append theme=LIGHT
or theme=DARK
to force a given theme.
Currency exchange rate parameter
This parameter will change the exchange rate of the currency. It only affects the front end and will not change the exchange rate on the dashboard (our backend).
This parameter is only available for the survey currency exchange rate.
Parameter | Possible values | Description |
---|---|---|
exchange | Float or Integer | This changes the currency exchange rate. Provide a Float or Integer which will be equal to 1 USD. Example: exchange = 1000 would mean 1 USD = 1000 currency. This parameter only works for surveys. |
Other parameters
parameter | possible values | description |
---|---|---|
hash | hmac sha1 hash from the URL | To validate the set parameter settings more information |
display_mode | surveys , offers ,gaming ,magic_receipts ,all , surveys,offers,... | Overrides which modes will be enabled. You can select between surveys, offers, games and combine them as you like. |
os | android , ios , desktop | Overrides the operating system that is currently used. |
sdk | IFRAME , TAB | Overrides where the offer wall is opened in an iframe or own tab |
in_app | true , false | Use this parameter when implementing the offer wall via a web view in mobile apps. |
username | "username123" | The username the user set on your page. This is used to display the username instead of anonymous in the leaderboard widget |
offer-id | id of an offer returned from our offer API | This parameter allows you to open a specific offer when visiting the offerwall. This can be used to redirect users from push notifications or deep links directly to our offerwall and a specific offer. |
maid | mobile advertising ID | This parameter sets the mobile advertising ID. It is a legacy parameter and not required anymore. |
Updated 3 days ago
You have now implemented BitLabs with your project. If you haven't done it already, it is time to configure server-to-server callbacks and the look and feel of your app.