BitLabs Flutter SDK
This page will guide you through the integration of our Flutter SDK into your app.
CHANGELOG
To check the latest version and all the previous ones of the SDK, you can check this page.
What's new in this page:
Since v2.2.0
- Deprecate Widget types implementation and add a new one.
- Deprecate Leaderboard Widget and add a new one.
Account Set-Up and retrieving your API Key
Before we can get started with implementing the SDK, you should retrieve your API Key for your integration from the BitLabs Dashboard. If you haven't created an account yet, you can do so here and follow the instructions for the dashboard starting with Sign up & Set up.
Adding SDK
In your pubspec file, add bitlabs
as a dependency.
dependencies:
bitlabs: ^2.1.0
Direct download
Alternatively, you can download the BitLabs Unity SDK by clicking here.
Initialision
You must initialise the SDK before you can use it. You can do so with the following code.
BitLabs.instance.init('YOUR_APP_TOKEN', 'USER_ID');
This is where you will need the API key from the dashboard, as you will need to replace "YOUR-TOKEN" with it. For "YOUR-USER-ID", you will need to dynamically insert the user id for each app user. Make sure the id used here is unique and that it does not change, as the user's profile will be stored under this id.
Support Localization
If want to support localization in the OfferWall, you have to add the LocalizationDelegate()
to your main App Widget as follows:
@override
Widget build(BuildContext context) {
return MaterialApp(
localizationsDelegates: [
...GlobalMaterialLocalizations.delegates,
LocalizationDelegate(), // This belongs to BitLabs and it's required for localization
],
supportedLocales: const [
Locale('en', ''),
Locale('es', ''),
Locale('de', ''),
Locale('fr', ''),
Locale('it', ''),
],
);
}
Using the SDK
Show BitLabs to the user
Now it's time to use the BitLabs SDK so that your users can start taking surveys. Call the .launchOfferWall()
function to open the Offer Wall/Direct Link. BitLabs will show up and the user will see qualifications or surveys.
BitLabs.instance.launchOfferWall(<BuildContext>);
The <BuildContext>
is that of the widget where BitLabs is visible in.
That's it!
Theoretically, this is all you have to do. Anything below is optional but can improve the user experience.
Check For Surveys - Check if there's something to do for the user
You can use .checkSurveys()
to check if a survey is available for the user. This function takes two callbacks as parameters: onResponse
with hasSurveys
, a bool which is true
whenever there's at least one survey or qualification question available for the user. Otherwise, it's false
. onFailure
with an Exception.
BitLabs.instance.checkSurveys(
(hasSurveys) => log('[Example] Checking Surveys -> '
'${hasSurveys ? 'Surveys Available!' : 'No Surveys!'}'),
(exception) => log('[Example] CheckSurveys $exception'),
);
Get Surveys Natively
You can use .getSurveys()
to get available surveys for the user. The onResponse
callback has a List.
BitLabs.instance.getSurveys(
(surveys) => log('[Example] Getting Surveys -> ${surveys.map((survey) => '
Survey ${survey.id} in ${survey.details.category.name}')}'),
(exception) => log('[Example] GetSurveys $exception'),
);
A Surveyhas the following properties:
Property | Type | Description |
---|---|---|
networkId | int | The id of the Network this survey belongs to. |
id | int | The id of the Survey. |
cpi | string | CPI of this survey in USD without any formatting applied. |
value | string | CPI formatted according to your app settings. Can be shown to the user directly. |
loi | double | Assumed length of the survey in minutes. |
remaining | int | Amount of users that can still open the survey |
details | Details(Category(name: string, iconUrl: string)) | The details of the category this Survey is classified in. |
rating | int | Difficulty ranking of this survey. 1-5 (1 = hard, 5 = easy). Minimum value is 1, maximum value is 5. |
link | string | This link can be used as is to open the survey. All relevant details are inserted on the server. |
missingQuestions | int? | The amount of questions that have to be answered before the survey is guaranteed to be openable by the user. |
Native Survey Widgets
getSurveyWidgets (DEPRECATED)
You can use .getSurveyWidgets()
to get a list of survey widgets. You can add them into a ListView as shown below.
ListView(
scrollDirection: Axis.horizontal,
children: BitLabs.instance.getSurveyWidgets(surveys, WidgetType.simple), // surveys here is a list of Survey objects
)
The WidgetType can be one of three options:

BitLabsWidget
You can add BitLabsWidget
widget into your widget tree wherever your want it to appear.
BitLabsWidget(
token: appToken,
uid: uid,
type: WidgetType.simple,
),
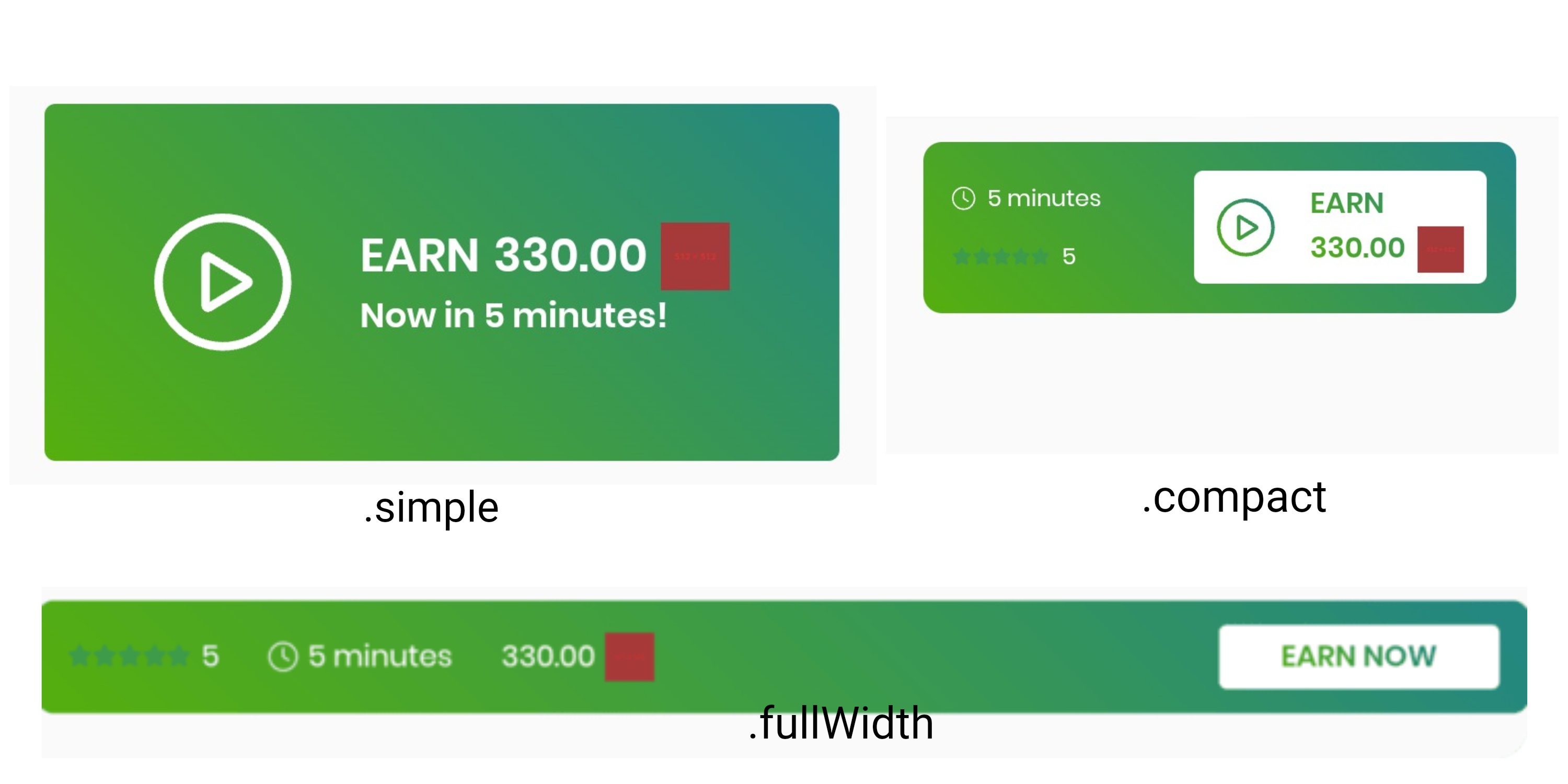
On Reward - Client Side Callbacks
You can use .setOnReward()
to set callback which receives the reward of the user and executes the behaviour you specify.
BitLabs.instance.setOnReward((reward) => {log('[Example] Reward for this session: $reward')});
This callback is invoked when the user leaves the Offer Wall, and the payout is the total reward the user got since the Offer Wall is opened until it is closed.
Important
Once you enable Offers in your BitLabs Dashboard, the Offerwall will be launched in Safari on iOS and thus you can only use server-to-server callbacks for reward tracking. This function will basically be useless in such a case.
For Android, the reward in this callback is just that of the surveys. For offers, please use the server-to-server callback.
We highly recommend using server-to-server callbacks instead! Please do not use this in apps where the user can withdraw real currency, as it might be exploitable.
However, if your app stores user data locally and does not sync with a server, this would be an option to still use BitLabs to reward your users.
Parameters
You can use .setTags()
to pass additional parameters to the OfferWall.
BitLabs.instance.setTags({'display_mode': 'offers', 'in_app': true});
Setting a new Map of Tags will replace the old ones.
Add a Parameter
Use addTag()
if you want to append new tags to an already existent Map of tags which you already set earlier.
BitLabs.instance.addTag('display_mode', 'offers');
BitLabs.instance.addTag('in_app', true);
Helpful
You can find a list of existing parameters here.
Request Tracking Authorisation - For the Advertising Id
Since Offers work best with a device's Ad Id, we will need to fetch that Id and send it to the offer wall. In iOS, this can only be done after the user gives authorisation. So, we created a method which asks the user for authorisation. You can have the discretion of when it's best to ask the user in your app and call this one method whenever you want.
BitLabs.instance.requestTrackingAuthorization()
IMPORTANT
In order to be able to show the Tracking Authorization request, you have to provide a custom text, known as a usage-description string, which displays in the system-permission alert request. Basically, just add this value to your Info.plist as shown in this official page.
Leaderboard
The Leaderboard widget shows your top 100 earning users and their corresponding earnings in your app's currency. And will open the Offerwall if clicked.
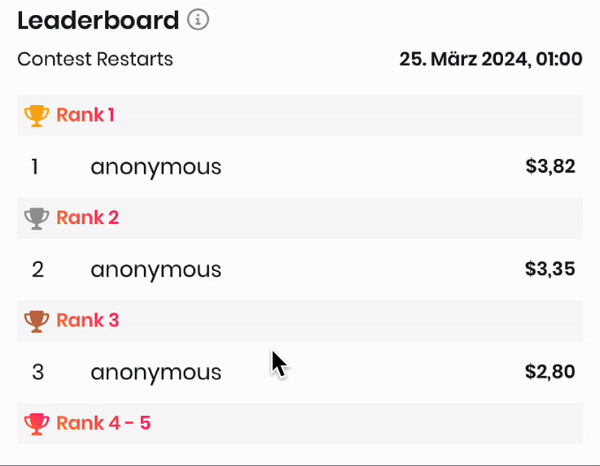
Reward
The rewards shown in the widget are custom to your app and will be paid by us to you and your users. By default these rewards are disabled and not shown so this widget only serves the purpose to display the best earning users. To enable the rewards and incentivise your users to do more surveys and offers in your app please contact us on: [email protected]
The earnings will reset at the end of each month and the top 100 users will get their corresponding reward. If a user is listed in the leaderboard it will see it's rank by the blue (You) besides the username.
All earnings and rewards in the leaderboard will be formatted as your app's settings set on our publisher dashboard.
Username
If you implement the leaderboard without updating your offerwall and widget integration all users will just be called anonymous. To pass us the correct usernames to your provided user id you will have to add a tag with key username before launching the offerwall.
Implementation
BitLabsLeaderboard (DEPRECATED)
To use the Leaderboard, after you initialise the BitLabs instance, you can simply add the BitLabsLeaderboard() widget to your widget tree.
@override
Widget build(Build context) {
return const BitLabsLeaderboard();
}
BitLabsWidget
Now, you use the BitLabsWidget with type
set as leaderboard
@override
Widget build(Build context) {
return BitLabsWidget(
token: appToken,
uid: uid,
type: WidgetType.leaderboard,
)
}
Gaming offers on iOS
To enable gaming offers for SDK integrations on iOS, you need to follow this guide.
Updated 5 months ago
You have now implemented BitLabs with your project. If you haven't done it already, it is time to configure server-to-server callbacks and the look and feel of your app.